断言
对象、数组、集合
ObjectUtils
StringUtils
CollectionUtil
文件、资源、IO 流
FileCopyUtils
ResourceUtils
StreamUtils
反射、AOP
ReflectionUtils
AopUtils
AopContext
最近发现同事写了不少重复的工具类,发现其中很多功能,Spring 自带的都有。于是整理了本文,希望能够帮助到大家!
断言
断言是一个逻辑判断,用于检查不应该发生的情况
Assert 关键字在 JDK1.4 中引入,可通过 JVM 参数-enableassertions开启
SpringBoot 中提供了 Assert 断言工具类,通常用于数据合法性检查
//要求参数object必须为非空(NotNull),否则抛出异常,不予放行 //参数 message 参数用于定制异常信息。 voidnotNull(Objectobject,Stringmessage) //要求参数必须空(Null),否则抛出异常,不予『放行』。 //和notNull()方法断言规则相反 voidisNull(Objectobject,Stringmessage) //要求参数必须为真(True),否则抛出异常,不予『放行』。 voidisTrue(booleanexpression,Stringmessage) //要求参数(List/Set)必须非空(NotEmpty),否则抛出异常,不予放行 voidnotEmpty(Collectioncollection,Stringmessage) //要求参数(String)必须有长度(即,NotEmpty),否则抛出异常,不予放行 voidhasLength(Stringtext,Stringmessage) //要求参数(String)必须有内容(即,NotBlank),否则抛出异常,不予放行 voidhasText(Stringtext,Stringmessage) //要求参数是指定类型的实例,否则抛出异常,不予放行 voidisInstanceOf(Classtype,Objectobj,Stringmessage) //要求参数`subType`必须是参数superType的子类或实现类,否则抛出异常,不予放行 voidisAssignable(ClasssuperType,ClasssubType,Stringmessage)
对象、数组、集合
ObjectUtils
获取对象的基本信息
//获取对象的类名。参数为 null 时,返回字符串:"null" StringnullSafeClassName(Objectobj) //参数为null时,返回0 intnullSafeHashCode(Objectobject) //参数为 null 时,返回字符串:"null" StringnullSafeToString(boolean[]array) //获取对象 HashCode(十六进制形式字符串)。参数为 null 时,返回0 StringgetIdentityHexString(Objectobj) //获取对象的类名和 HashCode。参数为 null 时,返回字符串:"" StringidentityToString(Objectobj) //相当于 toString()方法,但参数为 null 时,返回字符串:"" StringgetDisplayString(Objectobj)
判断工具
//判断数组是否为空 booleanisEmpty(Object[]array) //判断参数对象是否是数组 booleanisArray(Objectobj) //判断数组中是否包含指定元素 booleancontainsElement(Object[]array,Objectelement) //相等,或同为null时,返回true booleannullSafeEquals(Objecto1,Objecto2) /* 判断参数对象是否为空,判断标准为: Optional:Optional.empty() Array:length==0 CharSequence:length==0 Collection:Collection.isEmpty() Map:Map.isEmpty() */ booleanisEmpty(Objectobj)
其他工具方法
//向参数数组的末尾追加新元素,并返回一个新数组 A[]addObjectToArray(A[]array,Oobj) //原生基础类型数组-->包装类数组 Object[]toObjectArray(Objectsource)
StringUtils
字符串判断工具
//判断字符串是否为null,或""。注意,包含空白符的字符串为非空 booleanisEmpty(Objectstr) //判断字符串是否是以指定内容结束。忽略大小写 booleanendsWithIgnoreCase(Stringstr,Stringsuffix) //判断字符串是否已指定内容开头。忽略大小写 booleanstartsWithIgnoreCase(Stringstr,Stringprefix) //是否包含空白符 booleancontainsWhitespace(Stringstr) //判断字符串非空且长度不为0,即,NotEmpty booleanhasLength(CharSequencestr) //判断字符串是否包含实际内容,即非仅包含空白符,也就是NotBlank booleanhasText(CharSequencestr) //判断字符串指定索引处是否包含一个子串。 booleansubstringMatch(CharSequencestr,intindex,CharSequencesubstring) //计算一个字符串中指定子串的出现次数 intcountOccurrencesOf(Stringstr,Stringsub)
字符串操作工具
//查找并替换指定子串 Stringreplace(StringinString,StringoldPattern,StringnewPattern) //去除尾部的特定字符 StringtrimTrailingCharacter(Stringstr,chartrailingCharacter) //去除头部的特定字符 StringtrimLeadingCharacter(Stringstr,charleadingCharacter) //去除头部的空白符 StringtrimLeadingWhitespace(Stringstr) //去除头部的空白符 StringtrimTrailingWhitespace(Stringstr) //去除头部和尾部的空白符 StringtrimWhitespace(Stringstr) //删除开头、结尾和中间的空白符 StringtrimAllWhitespace(Stringstr) //删除指定子串 Stringdelete(StringinString,Stringpattern) //删除指定字符(可以是多个) StringdeleteAny(StringinString,StringcharsToDelete) //对数组的每一项执行trim()方法 String[]trimArrayElements(String[]array) //将URL字符串进行解码 StringuriDecode(Stringsource,Charsetcharset)
路径相关工具方法
//解析路径字符串,优化其中的“..” StringcleanPath(Stringpath) //解析路径字符串,解析出文件名部分 StringgetFilename(Stringpath) //解析路径字符串,解析出文件后缀名 StringgetFilenameExtension(Stringpath) //比较两个两个字符串,判断是否是同一个路径。会自动处理路径中的“..” booleanpathEquals(Stringpath1,Stringpath2) //删除文件路径名中的后缀部分 StringstripFilenameExtension(Stringpath) //以“.作为分隔符,获取其最后一部分 Stringunqualify(StringqualifiedName) //以指定字符作为分隔符,获取其最后一部分 Stringunqualify(StringqualifiedName,charseparator)
CollectionUtils
集合判断工具
//判断List/Set是否为空 booleanisEmpty(Collection>collection) //判断Map是否为空 booleanisEmpty(Map,?>map) //判断List/Set中是否包含某个对象 booleancontainsInstance(Collection>collection,Objectelement) //以迭代器的方式,判断List/Set中是否包含某个对象 booleancontains(Iterator>iterator,Objectelement) //判断List/Set是否包含某些对象中的任意一个 booleancontainsAny(Collection>source,Collection>candidates) //判断 List/Set 中的每个元素是否唯一。即 List/Set 中不存在重复元素 booleanhasUniqueObject(Collection>collection)
集合操作工具
//将Array中的元素都添加到List/Set中voidmergeArrayIntoCollection(Objectarray,Collection collection) //将Properties中的键值对都添加到Map中 voidmergePropertiesIntoMap(Propertiesprops,Map map) //返回List中最后一个元素 TlastElement(List list) //返回Set中最后一个元素 TlastElement(Set set) //返回参数candidates中第一个存在于参数source中的元素 EfindFirstMatch(Collection>source,Collection candidates) //返回 List/Set 中指定类型的元素。 TfindValueOfType(Collection>collection,Class type) //返回 List/Set 中指定类型的元素。如果第一种类型未找到,则查找第二种类型,以此类推 ObjectfindValueOfType(Collection>collection,Class>[]types) //返回List/Set中元素的类型 Class>findCommonElementType(Collectio>collection)
文件、资源、IO 流
FileCopyUtils
输入
//从文件中读入到字节数组中 byte[]copyToByteArray(Filein) //从输入流中读入到字节数组中 byte[]copyToByteArray(InputStreamin) //从输入流中读入到字符串中 StringcopyToString(Readerin)
输出
//从字节数组到文件 voidcopy(byte[]in,Fileout) //从文件到文件 intcopy(Filein,Fileout) //从字节数组到输出流 voidcopy(byte[]in,OutputStreamout) //从输入流到输出流 intcopy(InputStreamin,OutputStreamout) //从输入流到输出流 intcopy(Readerin,Writerout) //从字符串到输出流 voidcopy(Stringin,Writerout)
ResourceUtils
从资源路径获取文件
//判断字符串是否是一个合法的 URL 字符串。 staticbooleanisUrl(StringresourceLocation) //获取URL staticURLgetURL(StringresourceLocation) //获取文件(在JAR包内无法正常使用,需要是一个独立的文件) staticFilegetFile(StringresourceLocation)
Resource
//文件系统资源D:... FileSystemResource //URL资源,如file://...http://... UrlResource //类路径下的资源,classpth:... ClassPathResource //Web容器上下文中的资源(jar包、war包) ServletContextResource //判断资源是否存在 booleanexists() //从资源中获得File对象 FilegetFile() //从资源中获得URI对象 URIgetURI() //从资源中获得URI对象 URLgetURL() //获得资源的InputStream InputStreamgetInputStream() //获得资源的描述信息 StringgetDescription()
StreamUtils
输入
voidcopy(byte[]in,OutputStreamout) intcopy(InputStreamin,OutputStreamout) voidcopy(Stringin,Charsetcharset,OutputStreamout) longcopyRange(InputStreamin,OutputStreamout,longstart,longend)
输出
byte[]copyToByteArray(InputStreamin) StringcopyToString(InputStreamin,Charsetcharset) //舍弃输入流中的内容 intdrain(InputStreamin)
反射、AOP
ReflectionUtils
获取方法
//在类中查找指定方法 MethodfindMethod(Class>clazz,Stringname) //同上,额外提供方法参数类型作查找条件 MethodfindMethod(Class>clazz,Stringname,Class>...paramTypes) //获得类中所有方法,包括继承而来的 Method[]getAllDeclaredMethods(Class>leafClass) //在类中查找指定构造方法 ConstructoraccessibleConstructor(Class clazz,Class>...parameterTypes) //是否是equals()方法 booleanisEqualsMethod(Methodmethod) //是否是hashCode()方法 booleanisHashCodeMethod(Methodmethod) //是否是toString()方法 booleanisToStringMethod(Methodmethod) //是否是从Object类继承而来的方法 booleanisObjectMethod(Methodmethod) //检查一个方法是否声明抛出指定异常 booleandeclaresException(Methodmethod,Class>exceptionType)
执行方法
//执行方法 ObjectinvokeMethod(Methodmethod,Objecttarget) //同上,提供方法参数 ObjectinvokeMethod(Methodmethod,Objecttarget,Object...args) //取消 Java 权限检查。以便后续执行该私有方法 voidmakeAccessible(Methodmethod) //取消 Java 权限检查。以便后续执行私有构造方法 voidmakeAccessible(Constructor>ctor)
获取字段
//在类中查找指定属性 FieldfindField(Class>clazz,Stringname) //同上,多提供了属性的类型 FieldfindField(Class>clazz,Stringname,Class>type) //是否为一个"publicstaticfinal"属性 booleanisPublicStaticFinal(Fieldfield)
设置字段
//获取target对象的field属性值 ObjectgetField(Fieldfield,Objecttarget) //设置target对象的field属性值,值为value voidsetField(Fieldfield,Objecttarget,Objectvalue) //同类对象属性对等赋值 voidshallowCopyFieldState(Objectsrc,Objectdest) //取消 Java 的权限控制检查。以便后续读写该私有属性 voidmakeAccessible(Fieldfield) //对类的每个属性执行callback voiddoWithFields(Class>clazz,ReflectionUtils.FieldCallbackfc) //同上,多了个属性过滤功能。 voiddoWithFields(Class>clazz,ReflectionUtils.FieldCallbackfc, ReflectionUtils.FieldFilterff) //同上,但不包括继承而来的属性 voiddoWithLocalFields(Class>clazz,ReflectionUtils.FieldCallbackfc)
AopUtils
判断代理类型
//判断是不是Spring代理对象 booleanisAopProxy() //判断是不是jdk动态代理对象 isJdkDynamicProxy() //判断是不是CGLIB代理对象 booleanisCglibProxy()
获取被代理对象的 class
//获取被代理的目标class Class>getTargetClass()
AopContext
获取当前对象的代理对象
ObjectcurrentProxy()
-
数据
+关注
关注
8文章
7006浏览量
88951 -
spring
+关注
关注
0文章
340浏览量
14340
原文标题:别再自己瞎写工具类了,Spring Boot 内置工具类应有尽有, 建议收藏!!
文章出处:【微信号:AndroidPush,微信公众号:Android编程精选】欢迎添加关注!文章转载请注明出处。
发布评论请先 登录
相关推荐
Spring Boot如何实现异步任务
Spring Boot Starter需要些什么
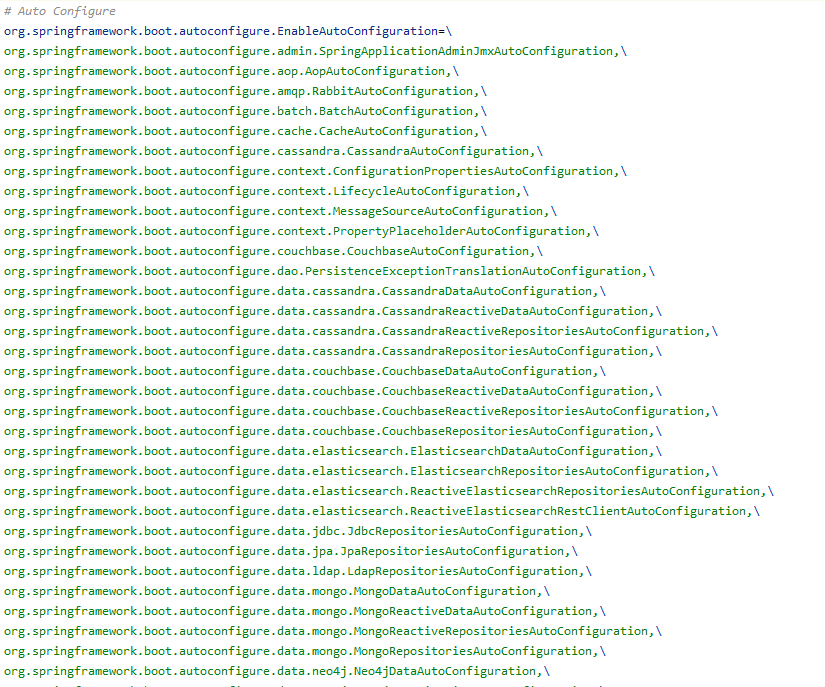
Spring Boot嵌入式Web容器原理是什么
Spring Boot框架错误处理
Spring Boot从零入门1 详述
Spring Boot特有的实践
强大的Spring Boot 3.0要来了
怎样使用Kiuwan保护Spring Boot应用程序呢?
Spring Boot Web相关的基础知识
Spring Boot Actuator快速入门
Spring Boot启动 Eureka流程
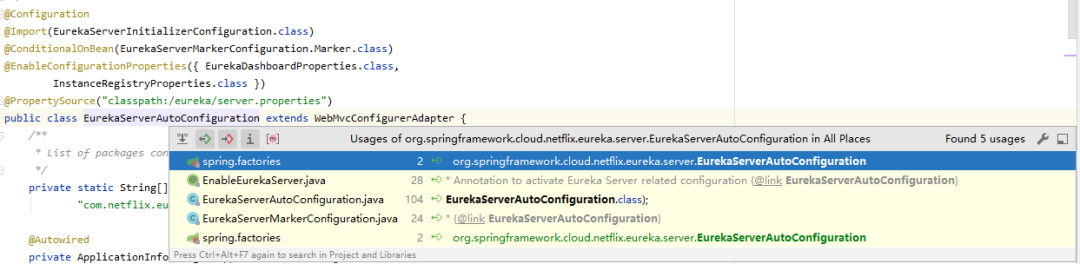
Spring Boot的启动原理
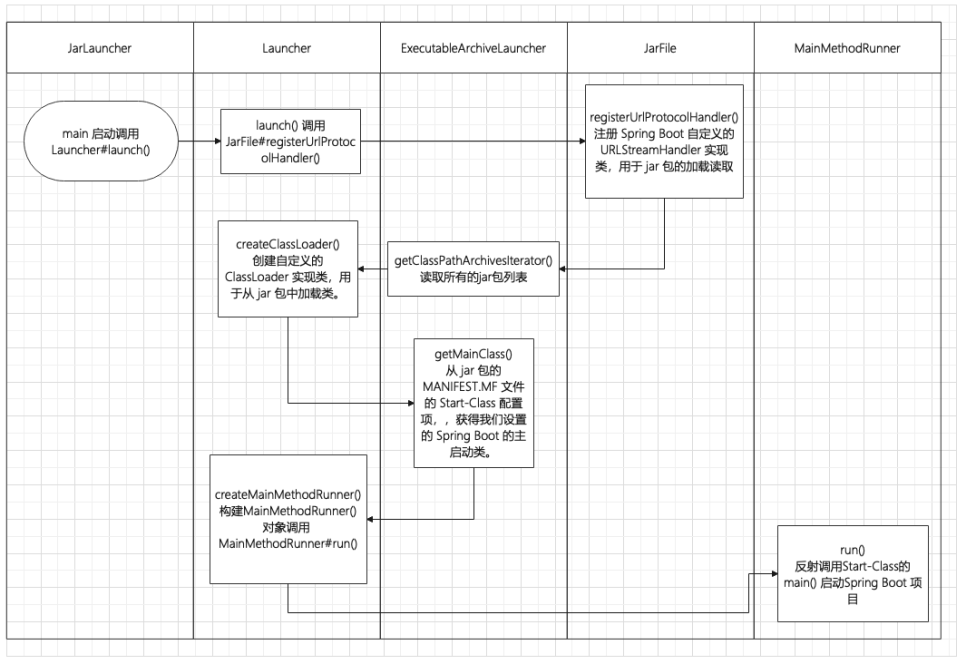
评论